Java Virtual Threads: A Deep Dive with Examples
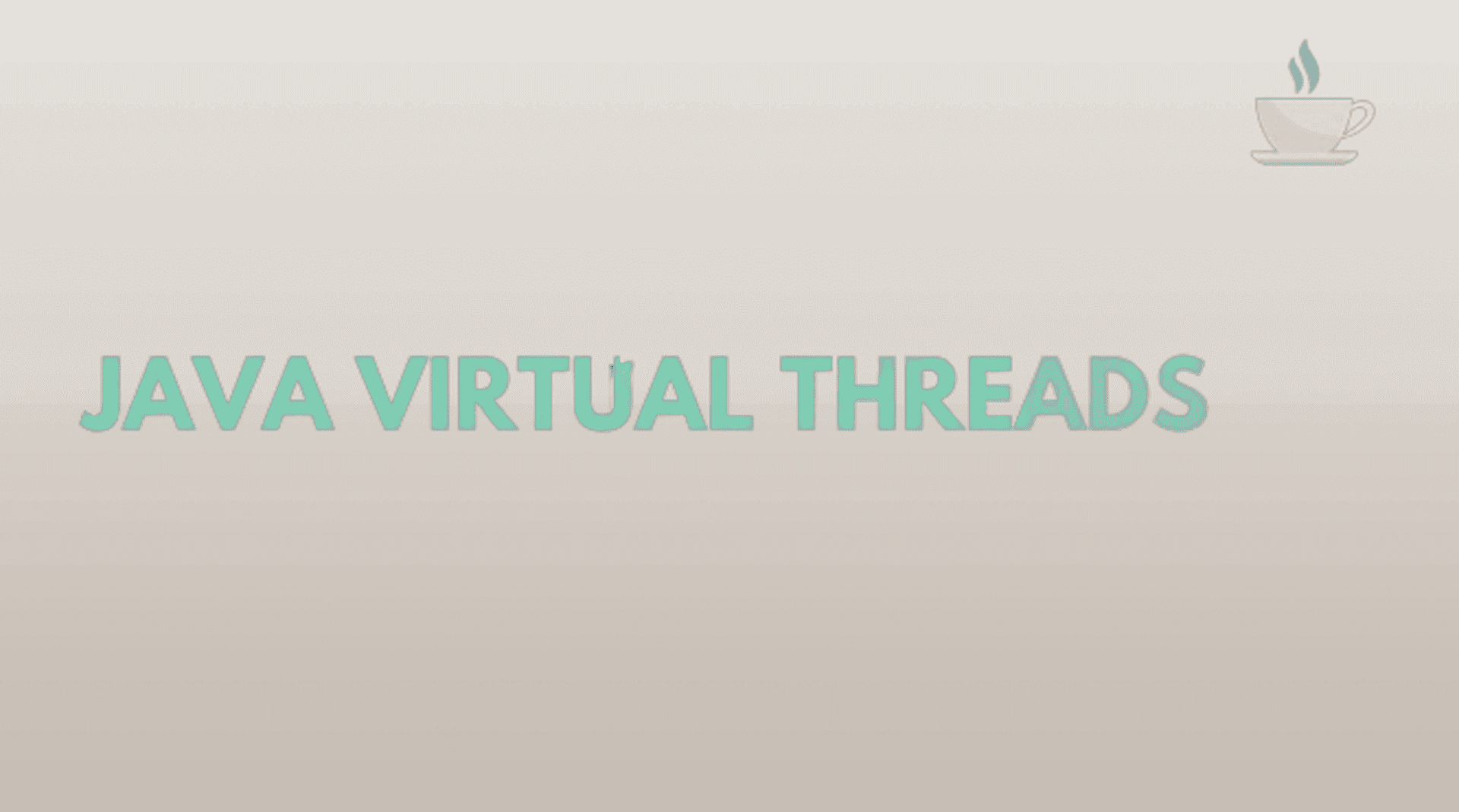
Content Table
- Understanding Java Virtual Threads
- What are Virtual Threads?
- Key Features of Virtual Threads
- Benefits of Java Virtual Threads
- Practical Examples of Java Virtual Threads
- Example 1: Basic Virtual Thread Creation
- Example 2: Virtual Threads in a Loop
- Example 3: Virtual Threads with CompletableFuture
- Advanced Usage and Considerations
- Handling Blocking I/O
- Monitoring and Debugging
- Conclusion
Java Virtual Threads, introduced as a preview feature in Java 19, promise to revolutionize concurrent programming in Java by simplifying the creation and management of threads. Unlike traditional threads, virtual threads are lightweight and optimized for high concurrency, making them an exciting development for Java developers. This article will delve into the concept of Java Virtual Threads, explore their benefits, and provide practical examples to illustrate their usage.
Understanding Java Virtual Threads
What are Virtual Threads?
Virtual threads, also known as Project Loom, aim to address the limitations of traditional Java threads by providing a lightweight alternative. Traditional threads, managed by the operating system, come with significant overhead in terms of memory and CPU usage, limiting the number of concurrent threads an application can efficiently manage. Virtual threads, on the other hand, are managed by the Java Virtual Machine (JVM) and are designed to be much more lightweight, allowing the creation of millions of concurrent threads without overwhelming system resources.
Key Features of Virtual Threads
- Lightweight: Virtual threads consume significantly less memory and CPU resources compared to traditional threads.
- Scalable Concurrency: They enable the creation of millions of concurrent threads, enhancing the scalability of applications.
- Simplified Thread Management: Developers can write code using traditional thread constructs without worrying about the overhead associated with traditional threads.
- Compatibility: Virtual threads are designed to be compatible with existing Java APIs and libraries, ensuring a smooth transition for developers.
Benefits of Java Virtual Threads
- Improved Resource Utilization: Virtual threads minimize the memory and CPU overhead associated with traditional threads, leading to better resource utilization and improved performance.
- Enhanced Scalability: With the ability to create millions of virtual threads, applications can handle a large number of concurrent tasks, making them more scalable.
- Simplified Concurrency: Developers can write concurrent code using familiar thread constructs, reducing the complexity of managing concurrency.
- Increased Productivity: The lightweight nature of virtual threads reduces the need for complex concurrency mechanisms, allowing developers to focus on business logic rather than thread management.
Practical Examples of Java Virtual Threads
Let's explore some practical examples to understand how virtual threads can be used in Java applications.
Example 1: Basic Virtual Thread Creation
public class VirtualThreadExample {
public static void main(String[] args) {
Thread virtualThread = Thread.ofVirtual().start(() -> {
System.out.println("Hello from a virtual thread!");
});
try {
virtualThread.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
In this example, a virtual thread is created using the Thread.ofVirtual().start()
method. The thread prints a message and then terminates. The join()
method ensures the main thread waits for the virtual thread to complete before exiting.
Example 2: Virtual Threads in a Loop
public class VirtualThreadsLoop {
public static void main(String[] args) {
for (int i = 0; i < 1000; i++) {
Thread.ofVirtual().start(() -> {
System.out.println("Task executed by virtual thread: " + Thread.currentThread().getName());
});
}
}
}
This example demonstrates the creation of multiple virtual threads in a loop. Each virtual thread executes a simple task, showcasing the ease of creating and managing a large number of concurrent tasks using virtual threads.
Example 3: Virtual Threads with CompletableFuture
import java.util.concurrent.CompletableFuture;
public class VirtualThreadsWithCompletableFuture {
public static void main(String[] args) {
CompletableFuture<Void> future = CompletableFuture.runAsync(() -> {
System.out.println("Task executed by virtual thread: " + Thread.currentThread().getName());
}, Thread.ofVirtual().factory());
future.join();
}
}
In this example, a virtual thread is used with CompletableFuture
to execute an asynchronous task. The Thread.ofVirtual().factory()
method is used to create a virtual thread factory, which is then passed to the runAsync
method.
Advanced Usage and Considerations
Handling Blocking I/O
Virtual threads are particularly useful for applications that involve blocking I/O operations, such as web servers and database clients. Traditional threads can become inefficient when blocked on I/O operations, but virtual threads handle these scenarios more gracefully.
import java.io.IOException;
import java.net.ServerSocket;
import java.net.Socket;
public class VirtualThreadServer {
public static void main(String[] args) throws IOException {
try (ServerSocket serverSocket = new ServerSocket(8080)) {
while (true) {
Socket clientSocket = serverSocket.accept();
Thread.ofVirtual().start(() -> handleClient(clientSocket));
}
}
}
private static void handleClient(Socket clientSocket) {
// Handle client connection
System.out.println("Handling client: " + clientSocket.getRemoteSocketAddress());
// Perform I/O operations with the client
}
}
In this example, a simple server application accepts client connections and handles them using virtual threads. Each client connection is processed in its own virtual thread, allowing the server to handle a large number of concurrent connections efficiently.
Monitoring and Debugging
While virtual threads offer numerous benefits, monitoring and debugging a large number of concurrent threads can be challenging. Developers should utilize tools and techniques such as thread dumps and logging to effectively monitor and debug virtual threads.
import java.lang.management.ManagementFactory;
import java.lang.management.ThreadMXBean;
public class VirtualThreadMonitoring {
public static void main(String[] args) {
ThreadMXBean threadMXBean = ManagementFactory.getThreadMXBean();
for (int i = 0; i < 1000; i++) {
Thread.ofVirtual().start(() -> {
System.out.println("Virtual thread running: " + Thread.currentThread().getName());
});
}
System.out.println("Total threads: " + threadMXBean.getThreadCount());
}
}
This example demonstrates how to monitor the total number of threads in the JVM using the ThreadMXBean
class. This can be useful for tracking the number of virtual threads in a running application.
Conclusion
Java Virtual Threads represent a significant advancement in concurrent programming, offering lightweight, scalable, and easy-to-use threads for modern Java applications. By reducing the overhead associated with traditional threads, virtual threads enable developers to build highly concurrent and efficient applications. As the Java ecosystem continues to evolve, virtual threads are poised to play a crucial role in shaping the future of Java development. Through practical examples, we've seen how virtual threads can be seamlessly integrated into Java applications, paving the way for more scalable and performant solutions.
That's all for this tutorial, I hope you enjoyed it, and until our next tutorial, take care.
Thank you!