How to upload a file to the AWS S3
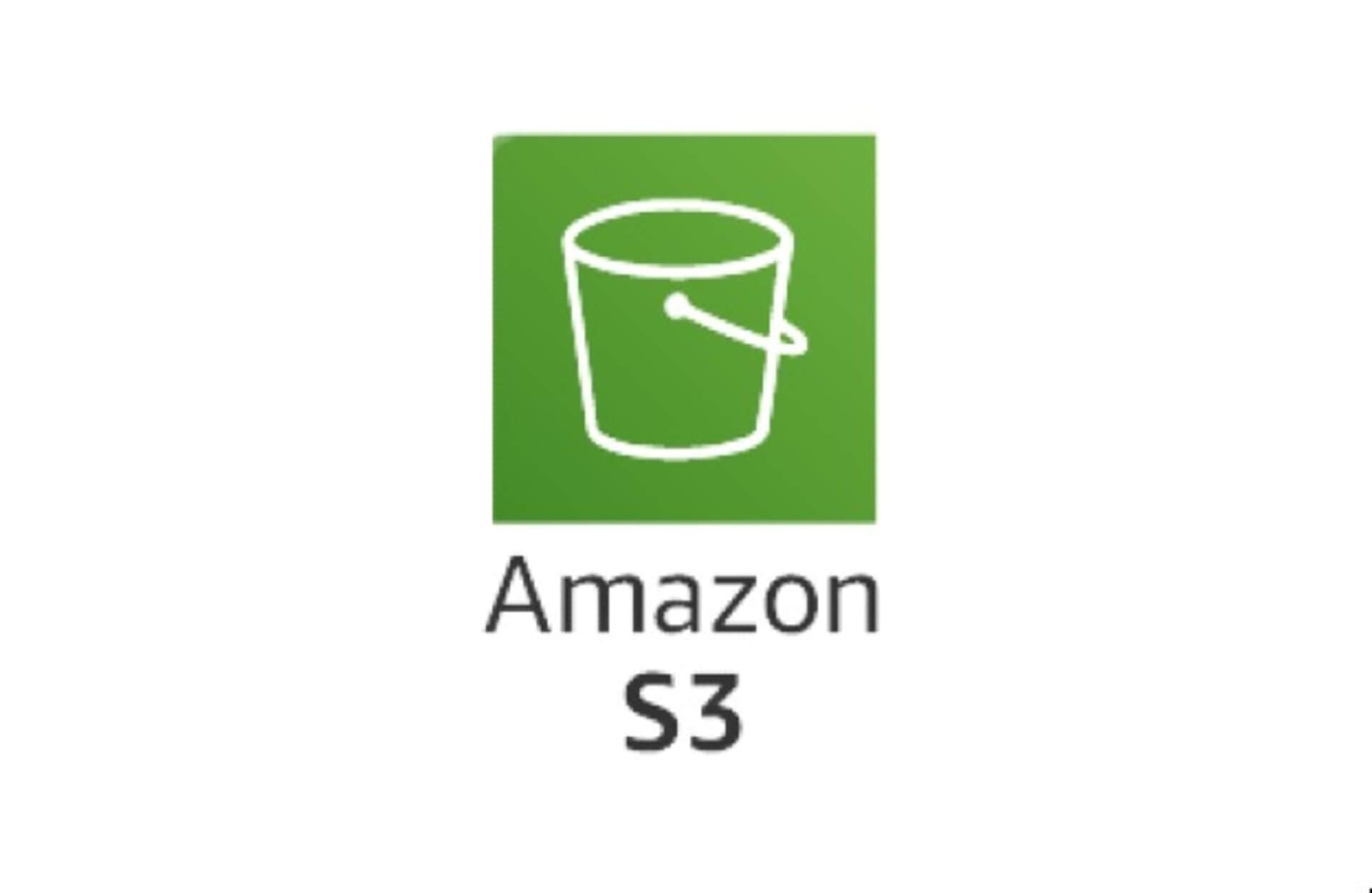
Amazon Simple Storage Service (Amazon S3) is a widely-used object storage service provided by Amazon Web Services (AWS). It is designed to store and retrieve any amount of data from anywhere on the web, making it a scalable and durable solution for managing data in the cloud. S3 provides developers and businesses with a secure and highly available storage infrastructure, allowing them to store and retrieve virtually unlimited amounts of data. In this tutorial we will see how to upload an xml file to S3 using AWS SDK and Java
Prerequisites
To Continue this tutorial, there are a some prerequisites that you need to fulfill:
- An AWS account to configure your AWS credentials.
- Install JDK 11 or higher
- Java Editor (Intellij or Eclipse)
In this tutorial, we'll upload an XML file to S3, so please make sure you've already created your bucket and generated the credentials.
Here's a step-by-step guide on how to upload an XML file to an S3 bucket :
Set up AWS Credentials
You need to provide your AWS credentials to interact with S3. This can be done using AWS Access Key ID and Secret Access Key or by using a configured AWS profile. You can set these up using the AWS CLI or by manually configuring them in the AWS SDK.
Add Dependencies
You should include the AWS SDK for Java in your project. You can add the necessary dependencies to your pom.xml
if you are using Maven or your build.gradle
if you are using Gradle. Here's an example for Maven:
<dependency>
<groupId>software.amazon.awssdk</groupId>
<artifactId>aws-sdk-java</artifactId>
<version>2.x.x</version>
</dependency>
implement the java Code to Upload XML File
Let's now implement the Java code to upload xml file to AWS S3 bucket.
import software.amazon.awssdk.auth.credentials.AwsBasicCredentials;
import software.amazon.awssdk.auth.credentials.StaticCredentialsProvider;
import software.amazon.awssdk.regions.Region;
import software.amazon.awssdk.services.s3.S3Client;
import software.amazon.awssdk.services.s3.model.PutObjectRequest;
import java.io.File;
public class S3Uploader {
public static void main(String[] args) {
// Replace with your AWS credentials
String accessKey = "YOUR_ACCESS_KEY";
String secretKey = "YOUR_SECRET_KEY";
String bucketName = "your-s3-bucket-name";
String objectKey = "your-xml-file.xml"; // Destination key in S3
String filePath = "path/to/your-local-xml-file.xml"; // Local XML file path
// Create AWS credentials provider
AwsBasicCredentials awsCreds = AwsBasicCredentials.create(accessKey, secretKey);
StaticCredentialsProvider credsProvider = StaticCredentialsProvider.create(awsCreds);
// Initialize S3 client
S3Client s3Client = S3Client.builder()
.region(Region.US_EAST_1) // Replace with your desired AWS region
.credentialsProvider(credsProvider)
.build();
// Upload XML file to S3
s3Client.putObject(PutObjectRequest.builder()
.bucket(bucketName)
.key(objectKey)
.build(),
new File(filePath).toPath());
System.out.println("XML file uploaded to S3");
// Close the S3 client
s3Client.close();
}
}
Make sure to replace YOUR_ACCESS_KEY
and YOUR_SECRET_KEY
with your actual AWS access key and secret key. Also, update the bucketName
, objectKey
, filePath
, and the desired AWS region.
This code will upload the specified XML file to the specified S3 bucket. Ensure that your AWS credentials have appropriate permissions to write to the S3 bucket.
That's all for this tutorial, I hope you enjoyed it, and until our next tutorial, happy uploading and take care.
Thank you!