Spring Boot: Features, Benefits, and Code Examples
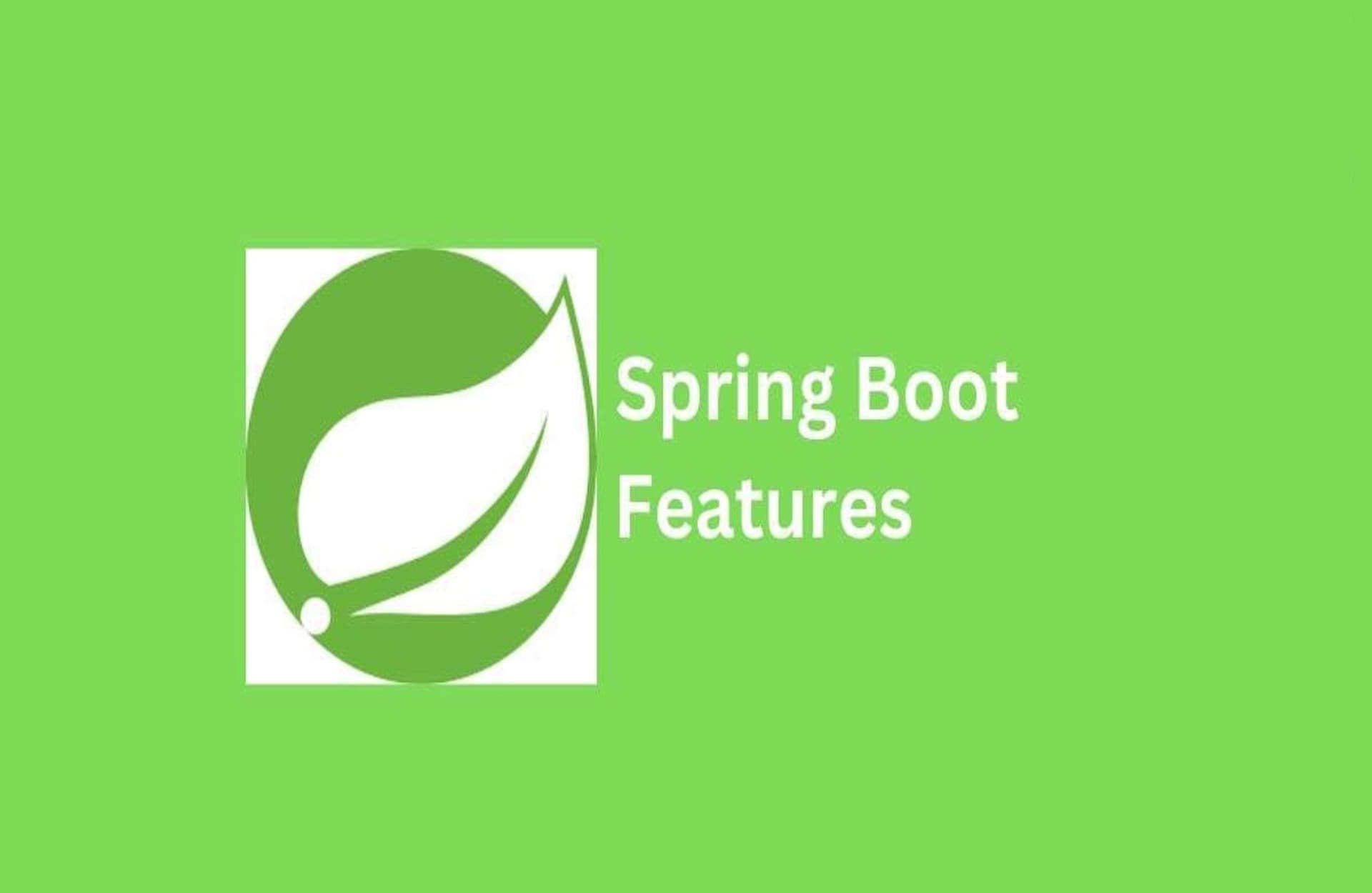
Content Table
- What is Spring Boot?
- Why Choose Spring Boot?
- Spring Boot Features
- 1. Auto-Configuration
- How It Works
- Example: Autoconfigured REST Controller
- 2. Starter Dependencies
- Common Starter Dependencies
- Example: Dependency Management
- 3. Embedded Servers
- Advantages
- Customization
- 4. Spring Boot Actuator
- Key Endpoints
- Setup
- 5. Externalized Configuration
- Properties Example
- YAML Example
- Accessing Configuration
- 6. DevTools
- Setup
- Features
- 7. Spring Profiles
- Setup Profiles
- 8. Security
- Basic Authentication
- 9. Testing Support
- Conclusion
Spring Boot is a groundbreaking framework that simplifies Java application development. It builds upon the Spring Framework, providing an opinionated approach to configuration and setup, which saves developers time and effort. Whether you're building microservices, RESTful APIs, or complex enterprise applications, Spring Boot is a go-to framework for Java developers.
In this article, we’ll delve deeply into Spring Boot's core features, explore its use cases, and provide hands-on examples to demonstrate its capabilities.
What is Spring Boot?
Spring Boot is an extension of the Spring Framework. It was introduced to address the complexity of manually configuring Spring applications, especially for large-scale projects. By offering default configurations, embedded servers, and production-ready tools, Spring Boot eliminates boilerplate code and simplifies application setup.
Why Choose Spring Boot?
- Ease of Use: Start projects quickly with minimal setup.
- Reduced Complexity: Spring Boot auto-configures most components, sparing you from writing lengthy configuration files.
- Production-Ready: Built-in features like health checks, metrics, and externalized configuration make Spring Boot a top choice for production environments.
- Microservices Support: Ideal for creating modular, independently deployable microservices.
- Extensive Ecosystem: Integrates seamlessly with the Spring ecosystem, including Spring Security, Spring Data, and Spring Cloud.
Spring Boot Features
Here’s a detailed look at Spring Boot’s most prominent features:
1. Auto-Configuration
Spring Boot’s autoconfiguration feature is its crown jewel. It automatically configures application components based on the dependencies present in your project’s classpath.
How It Works
When Spring Boot starts, it scans the classpath for dependencies and configures beans accordingly. For instance:
- If
spring-boot-starter-web
is included, it sets up a web server, aDispatcherServlet
, and default error handling. - If
spring-data-jpa
is included, it configures a JPAEntityManagerFactory
.
Example: Autoconfigured REST Controller
Here’s a simple REST application that works out-of-the-box with Spring Boot’s autoconfiguration:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
@RestController
class GreetingController {
@GetMapping("/greet")
public String greet() {
return "Hello, Spring Boot!";
}
}
With spring-boot-starter-web
in your dependencies, the application automatically configures an embedded Tomcat server and sets up GreetingController
as a REST controller.
2. Starter Dependencies
Spring Boot provides “starters” — curated dependency bundles for common use cases. These reduce the complexity of managing multiple dependencies and their versions.
Common Starter Dependencies
spring-boot-starter-web
: For building web applications.spring-boot-starter-data-jpa
: For database access using JPA and Hibernate.spring-boot-starter-security
: For authentication and authorization.spring-boot-starter-test
: For unit and integration testing.
Example: Dependency Management
Add the following to your pom.xml
for a RESTful web application:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
This single dependency brings in:
- Spring MVC
- Embedded Tomcat
- Jackson (for JSON processing)
- Logging libraries
3. Embedded Servers
Spring Boot eliminates the need to deploy WAR files to external servers by bundling embedded servers like Tomcat, Jetty, and Undertow.
Advantages
- Applications are self-contained, making them easy to deploy.
- Simplifies the CI/CD pipeline as no separate server setup is required.
Customization
You can configure the embedded server via application.properties
or programmatically.
Example: Changing the Port
server.port=9090
Programmatically:
import org.springframework.boot.web.server.ConfigurableWebServerFactory;
import org.springframework.boot.web.server.WebServerFactoryCustomizer;
import org.springframework.stereotype.Component;
@Component
public class ServerCustomizer implements WebServerFactoryCustomizer<ConfigurableWebServerFactory> {
@Override
public void customize(ConfigurableWebServerFactory factory) {
factory.setPort(9090);
}
}
4. Spring Boot Actuator
Actuator is a powerful feature that provides endpoints for monitoring and managing applications in production. It integrates seamlessly with monitoring tools like Prometheus and Grafana.
Key Endpoints
/actuator/health
: Displays application health status./actuator/metrics
: Shows performance metrics./actuator/env
: Exposes environment properties.
Setup
Add the Actuator dependency:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
Enable specific endpoints:
management.endpoints.web.exposure.include=health,metrics
Access the endpoints at:
http://localhost:8080/actuator/health
5. Externalized Configuration
Spring Boot supports configuration through properties, YAML files, environment variables, and command-line arguments. This feature promotes flexibility across environments (e.g., development, staging, production).
Properties Example
server.port=8081
spring.application.name=MyApp
YAML Example
server:
port: 8081
spring:
application:
name: MyApp
Accessing Configuration
Bind external properties to Java beans using @ConfigurationProperties
.
Example:
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.stereotype.Component;
@Component
@ConfigurationProperties(prefix = "app")
public class AppConfig {
private String name;
private String version;
// Getters and Setters
}
application.yml
:
app:
name: MyApplication
version: 1.0
6. DevTools
Spring Boot DevTools enhances developer productivity by enabling features like hot-reloading, live templates, and automatic restart during development.
Setup
Add DevTools dependency:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
</dependency>
Features
- Hot Reloading: Automatically restarts the application on code changes.
- Property Defaults: Disables caching for better development experience.
7. Spring Profiles
Profiles enable different configurations for different environments. For example, you might use an in-memory database for development and a production database for deployment.
Setup Profiles
Define environment-specific configurations:
application-dev.yml
:
spring:
datasource:
url: jdbc:h2:mem:devdb
application-prod.yml
:
spring:
datasource:
url: jdbc:mysql://prod-db:3306/proddb
Activate profiles using:
spring.profiles.active=dev
8. Security
Spring Boot simplifies the integration of Spring Security, making it easy to secure your applications.
Basic Authentication
Add spring-boot-starter-security
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
By default, Spring Security applies basic authentication. You can customize it using a SecurityConfig
class.
9. Testing Support
Spring Boot provides extensive support for testing, including:
- Mocking dependencies using
@MockBean
. - Integration testing with
@SpringBootTest
. - Testing REST endpoints with
MockMvc
.
Example Test:
import org.junit.jupiter.api.Test;
import org.springframework.boot.test.context.SpringBootTest;
@SpringBootTest
class DemoApplicationTests {
@Test
void contextLoads() {
}
}
Conclusion
Spring Boot is a comprehensive framework that streamlines Java application development. Its powerful features like autoconfiguration, embedded servers, Actuator, and profiles make it a versatile tool for building modern applications. Whether you're developing microservices, REST APIs, or enterprise systems, Spring Boot offers the tools and flexibility to meet your needs. By mastering these features and applying them effectively, you can build scalable, production-grade applications with ease.
That's all for this tutorial, I hope you enjoyed it, and until our next tutorial, happy uploading and take care.
Thank you!