Set up the redux toolkit in the react application
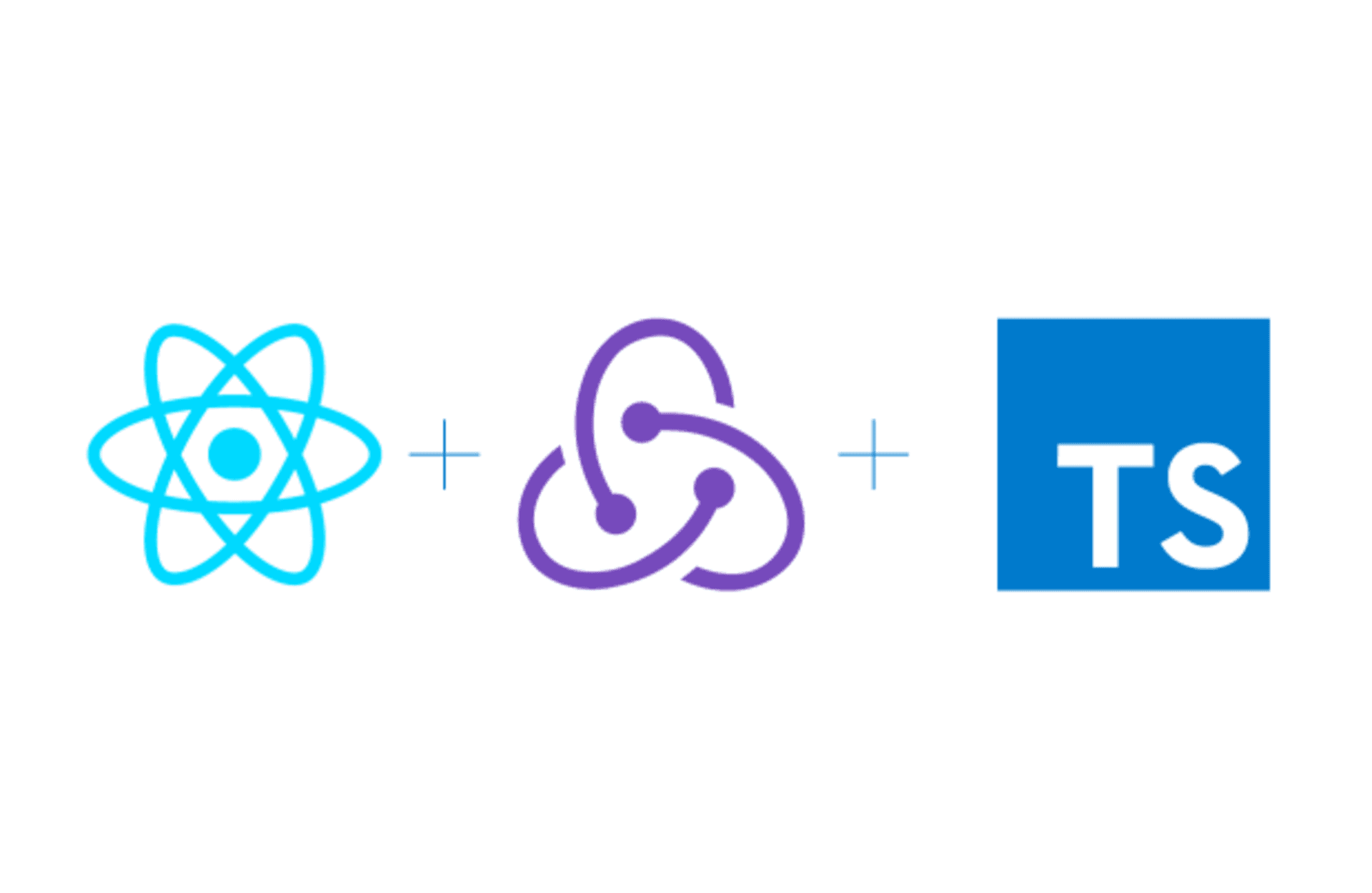
In the world of web development, Redux has become a popular choice for managing state in JavaScript applications. With its predictable and centralized state management, Redux offers a reliable solution for handling complex data flows. However, setting up Redux in a React and TypeScript project can be a daunting task, especially for developers who are new to these technologies.
In this article, we will guide you step by step on how to set up the Redux Toolkit with React and TypeScript. By following this tutorial, you will be equipped with the knowledge and tools necessary to integrate Redux into your projects seamlessly. So, let's get started and unlock the power of Redux in your web development journey!
Understanding Redux Toolkit and its Benefits
Before diving into the step-by-step process of setting up Redux Toolkit with React and TypeScript, it's essential to understand what Redux Toolkit is and the benefits it offers.
Redux Toolkit is the official recommended way to write Redux logic. It provides a set of utilities and abstractions that simplify the process of creating Redux stores, writing reducers, and dispatching actions. With the inclusion of the Redux Toolkit in your project, you can significantly reduce boilerplate code and make your Redux codebase more maintainable and scalable.
Some of the key benefits of using Redux Toolkit include:
-
Simplified code: Redux Toolkit simplifies the process of writing Redux logic by providing concise abstractions, such as
createSlice
, which combines actions and reducers into a single module. -
Immutable updates: Redux Toolkit uses the immer library by default, allowing you to write reducers that directly modify the state without worrying about immutability.
-
Built-in devtools integration: Redux Toolkit includes the Redux DevTools Extension preconfigured, giving you a powerful debugging tool out of the box.
-
Enhanced performance: Redux Toolkit optimizes the generated Redux store, resulting in improved performance and reduced memory consumption.
By leveraging these benefits, you can streamline your Redux setup and enhance your productivity as a developer. In the next section, we will delve into the step-by-step process of setting up Redux Toolkit with React and TypeScript, so stay tuned!
Setting Up React and TypeScript
Setting up React and TypeScript is the first step towards utilizing Redux Toolkit in your project.
To get started, ensure you have Node.js and npm (Node Package Manager) installed on your machine. If not, you can download and install them from the official Node.js website.
Once you have the necessary tools, open your terminal and navigate to the root directory of your project. Create a new React app by running the following command:
npx create-react-app my-redux-app --template typescript
cd my-redux-app
This command will create a new React app using TypeScript as the default template. It will also install all the required dependencies for a React project.
Installing Redux Toolkit
To install Redux Toolkit, open your terminal and navigate to the root directory of your project (the same directory where you previously set up React and TypeScript). Now, run the following command to install Redux Toolkit as a dependency:
npm install @reduxjs/toolkit react-redux
Once the installation is complete, you can verify that Redux Toolkit has been successfully installed by checking the "dependencies" section in your project's package.json file.
Next, let's move on to configuring Redux Toolkit with your React and TypeScript project in the following section.
Configuring Redux Store
Now that Redux Toolkit is installed in your project, it's time to configure the Redux store.
To create a Redux store using Redux Toolkit, you need to import the configureStore
function from the @reduxjs/toolkit
package.
In your project's root directory, navigate to the file where you want to set up your Redux store. Typically, this file is named store.ts
Import configureStore
at the top of your file:
import { configureStore } from '@reduxjs/toolkit';
Next, define your initial state and reducers. Redux Toolkit provides a convenient way to define them using the createSlice
function.
Create a new file named counterSlice.ts
(you can name it whatever you like) in the same directory as your store file. In counterSlice.ts
, define your initial state, reducers, and any other additional logic specific to your application.
In your store file, import your counterSlice
and any other slices you created:
import counterSlice from './counterSlice';
Now, you can configure your Redux store using the configureStore
function. Pass an object containing your slices as an argument to configureStore
:
Finally, export your Redux store from the store file:
// src/app/store.ts
import { configureStore } from '@reduxjs/toolkit';
import counterSlice from './counterSlice';
const store = configureStore({
reducer: {
counter: counterSlice.reducer,
// Add other slices here if you have created them
},
});
export type RootState = ReturnType<typeof store.getState>;
export type AppDispatch = typeof store.dispatch;
export default store;
Congratulations! You have successfully configured your Redux store using Redux Toolkit with React and TypeScript. In the next section, we will discuss how to use Redux Toolkit's useDispatch
and useSelector
hooks to interact with the Redux store.
Creating Actions and Reducers
Now that we have our Redux store set up using Redux Toolkit, let's move on to creating actions and reducers.
Actions are payloads of information that send data from your application to the Redux store. Reducers specify how the application's state changes in response to these actions.
In Redux Toolkit, actions and reducers are conveniently created using the createSlice
function. This function generates reducer functions automatically based on the provided initial state and a set of "slice" reducer functions.
Inside your counterSlice.ts
file, add the following code:
import { createSlice, PayloadAction } from '@reduxjs/toolkit';
interface CounterState {
value: number;
}
const initialState: CounterState = {
value: 0,
};
const counterSlice = createSlice({
name: 'counter',
initialState,
reducers: {
increment: (state) => {
state.value++;
},
decrement: (state) => {
state.value--;
},
incrementByAmount: (state, action: PayloadAction<number>) => {
state.value += action.payload;
},
},
});
export const { increment, decrement, incrementByAmount } = counterSlice.actions;
export default counterSlice.reducer;
In the code above, we define the CounterState
interface to represent the shape of our state object. The initialState
sets the initial value of the value
property.
The createSlice
function generates the action and reducer functions for us. The name
parameter specifies the name of the slice, while the reducers
object contains our slice reducer functions. Each function modifies the state object directly.
Finally, we export the generated action functions (increment
, decrement
, incrementByAmount
) and the reducer function from our slice.
In the next section, we will explore how to use these actions and reducers to update the state in our React components.
Connect Redux Store to the App
After adding creating Actions and Reducers, let's see how to connect the redux store to the application.
The most common way to do this is to wrap your app with the Provider
from react-redux
in src/index.tsx
as shown in the example below:
// src/index.tsx
import React from 'react';
import ReactDOM from 'react-dom';
import { Provider } from 'react-redux';
import App from './App';
import store from './app/store';
ReactDOM.render(
<Provider store={store}>
<App />
</Provider>,
document.getElementById('root')
);
Use Redux in Components
You can now use Redux in your components. For example, in a component file (src/components/Counter.tsx) add the following code:
// src/components/Counter.tsx
import React from 'react';
import { useDispatch, useSelector } from 'react-redux';
import { RootState } from '../app/store';
import { increment, decrement } from '../features/counterSlice';
const Counter: React.FC = () => {
const dispatch = useDispatch();
const count = useSelector((state: RootState) => state.counter.value);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => dispatch(increment())}>Increment</button>
<button onClick={() => dispatch(decrement())}>Decrement</button>
</div>
);
};
export default Counter;
When the user clicks on the Increment
button the action increment()
gets dispatched which will update the counter state (increments the value by 1) and the same thing when the user clicks on the Decrement
button.
Testing and Debugging Redux Toolkit
Testing and debugging are crucial aspects of developing any application, and Redux Toolkit provides excellent support for both. In this section, we will discuss how to test and debug Redux Toolkit code in your React and TypeScript application.
When it comes to testing, Redux Toolkit makes it easier by providing utilities like configureStore
and createSlice
that are easily testable. You can write unit tests for your reducers, action creators, and middleware using popular testing libraries like Jest and Vitest.
To debug your Redux Toolkit code, you can leverage Redux DevTools Extension. This extension allows you to inspect the state of your Redux store, track actions, and travel back and forth in time to debug issues. Additionally, Redux Toolkit provides helpful error messages and warnings in development mode to aid in debugging.
In the next section, we will explore some best practices for organizing your Redux Toolkit code and further optimize your Redux setup. Stay tuned for more valuable insights!
Conclusion
In this blog series, we have covered the step-by-step process of setting up Redux Toolkit with React and TypeScript. We discussed the benefits of using Redux Toolkit and how it simplifies state management in your applications.
That's all for this article, I hope you enjoyed it, and until our next article, take care.
Thank you!