Migration to Spring Boot 3 guide
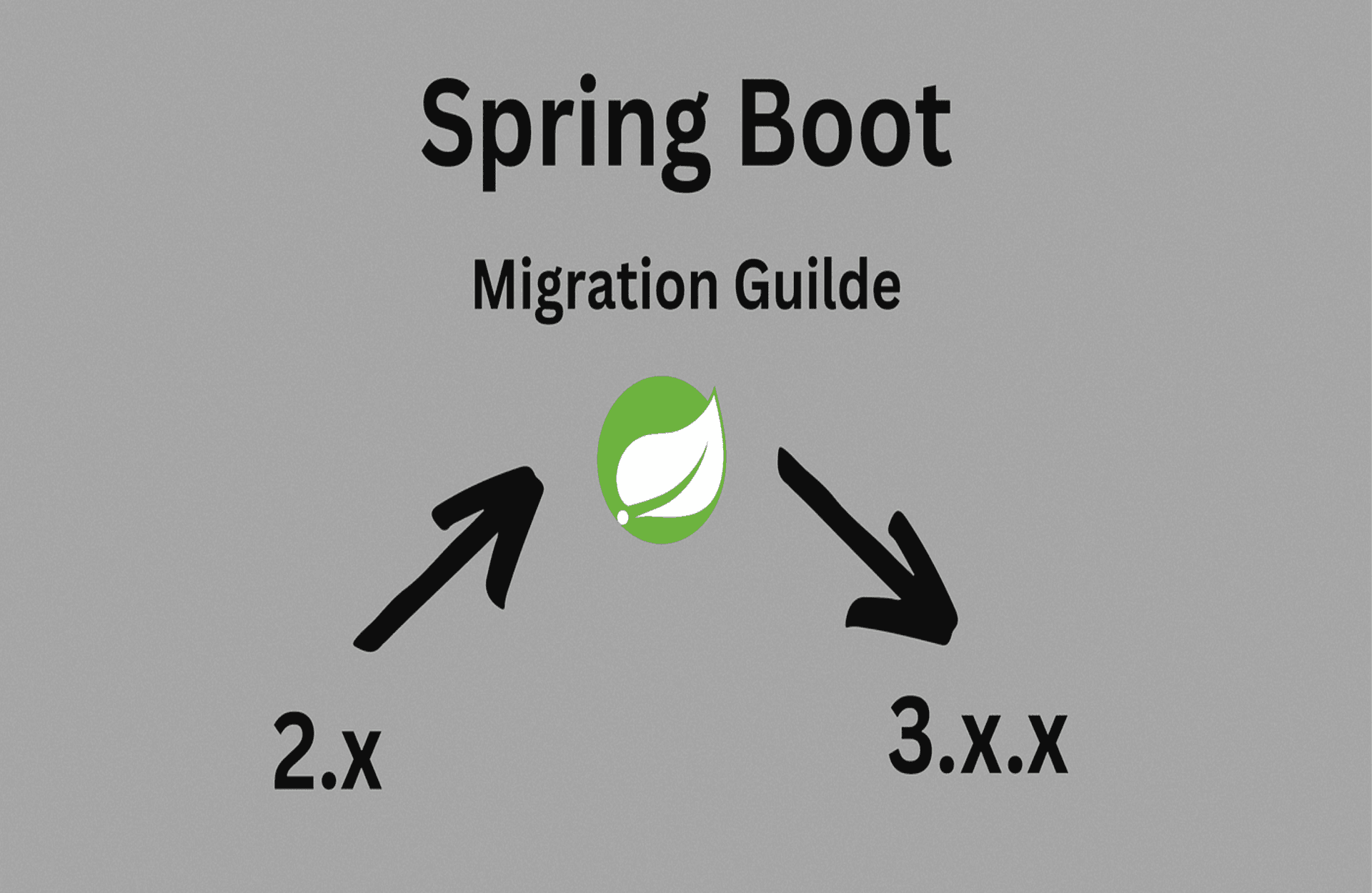
Content Table
- Why Migrate to Spring Boot 3?
- Key Changes in Spring Boot 3
- 1. Jakarta EE Migration
- 2. Native Image Support with GraalVM
- 3. Observability and Micrometer Integration
- 4. Java 17 Baseline
- 5. Updated Dependency Management
- Step-by-Step Migration Process
- 1. Assess Your Current Application
- 2. Update Dependencies
- 3. Refactor Namespace Changes
- 4. Test and Validate
- 5. Deploy to Staging
- Best Practices for Migration
- Conclusion
Spring Boot has been a reliable and powerful framework for Java developers, and with the release of Spring Boot 3, there are exciting new features and significant changes to consider. This version brings improved performance, enhanced native support, and alignment with Jakarta EE standards. However, migrating to Spring Boot 3 requires careful planning to ensure a smooth transition while leveraging its new capabilities.
This guide provides a comprehensive roadmap for migrating your application to Spring Boot 3, exploring key changes, practical examples, and best practices.
Why Migrate to Spring Boot 3?
Upgrading to Spring Boot 3 offers numerous advantages:
-
Jakarta EE 10 Support:
- Transition from the
javax.*
namespace tojakarta.*
, ensuring compatibility with modern Java standards.
- Transition from the
-
Enhanced Native Support:
- Optimized for GraalVM native images, enabling faster startup times and reduced resource usage.
-
Baseline Java 17:
- Leverage modern Java features like pattern matching, records, and improved performance.
-
Improved Observability:
- Seamless integration with observability tools via Micrometer, including metrics and tracing.
-
Long-Term Support:
- Access to updates, bug fixes, and a future-proof platform.
Key Changes in Spring Boot 3
1. Jakarta EE Migration
The most notable change is the migration from javax.*
to jakarta.*
namespaces. This shift reflects the evolution of Java EE into Jakarta EE under the Eclipse Foundation.
Code Changes:
- Replace
javax.persistence.Entity
withjakarta.persistence.Entity
. - Update validation annotations, e.g.,
javax.validation.constraints.NotNull
becomesjakarta.validation.constraints.NotNull
.
Example Update:
// Before
import javax.persistence.Entity;
import javax.validation.constraints.NotNull;
// After
import jakarta.persistence.Entity;
import jakarta.validation.constraints.NotNull;
2. Native Image Support with GraalVM
Spring Boot 3 enhances native image compatibility for applications running in cloud and serverless environments.
Setup for Native Images:
- Add the AOT (Ahead-of-Time) processing plugin:
Maven Configuration:
<plugin>
<groupId>org.springframework.experimental</groupId>
<artifactId>spring-aot-maven-plugin</artifactId>
<version>0.12.0</version>
<executions>
<execution>
<goals>
<goal>generate</goal>
</goals>
</execution>
</executions>
</plugin>
- Build the native image using GraalVM:
mvn spring-boot:build-image
- Optimize reflection configurations if needed for third-party libraries.
3. Observability and Micrometer Integration
Observability is a first-class feature in Spring Boot 3. Micrometer provides robust tools for metrics collection, distributed tracing, and logging.
New Features:
- Automatic instrumentation for HTTP requests, databases, and caches.
- Out-of-the-box support for Prometheus, Grafana, and OpenTelemetry.
Setup Example:
Add the Micrometer dependencies to your pom.xml
:
<dependency>
<groupId>io.micrometer</groupId>
<artifactId>micrometer-registry-prometheus</artifactId>
</dependency>
Enable metrics in application.properties
:
management.endpoints.web.exposure.include=metrics,health
management.metrics.export.prometheus.enabled=true
4. Java 17 Baseline
Spring Boot 3 requires Java 17 or later. This allows developers to benefit from:
- Improved garbage collection with G1 and ZGC.
- Language features like pattern matching, records, and text blocks.
- Enhanced security with the latest JVM updates.
Tip: Use tools like jenv
or Docker to manage Java versions.
5. Updated Dependency Management
Ensure all your dependencies are compatible with your Spring Boot 3 version. Update your pom.xml
or build.gradle
to include the correct version:
Maven Configuration
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>3.2.2</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
Gradle Configuration
dependencies {
implementation platform("org.springframework.boot:spring-boot-dependencies:3.2.2")
}
Step-by-Step Migration Process
1. Assess Your Current Application
Begin by evaluating your existing application:
- Identify dependencies and their versions.
- Check for
javax.*
usage in your codebase. - Review configurations in
application.properties
orapplication.yml
. - Ensure you are using Java 17 or later.
Tooling Tip: Use mvn dependency:tree
to list your project’s dependencies.
2. Update Dependencies
Upgrade all dependencies to their Spring Boot 3.X.X-compatible versions.
Common Updates:
- Hibernate: Ensure compatibility with Jakarta EE.
- Spring Data: Use the latest version aligned with your Spring Boot 3.X.X version.
- Security: Update Spring Security configurations to reflect namespace changes.
3. Refactor Namespace Changes
Replace all javax.*
imports with jakarta.*
equivalents. Use IDE refactoring tools to expedite the process.
Example Update:
// Before
import javax.servlet.http.HttpServletRequest;
// After
import jakarta.servlet.http.HttpServletRequest;
4. Test and Validate
Run your test suite to identify breaking changes:
- Unit Tests: Validate core functionality.
- Integration Tests: Check interactions with databases, APIs, and services.
- Performance Tests: Measure startup times and memory usage.
Use tools like JUnit 5 and Testcontainers to simulate production-like environments.
5. Deploy to Staging
Deploy the migrated application to a staging environment to verify behavior under realistic conditions. Monitor logs, metrics, and traces for anomalies.
Best Practices for Migration
- Start Small
Migrate one module or microservice at a time to minimize risks and identify potential issues early.
- Automate Tests
Invest in automated testing to catch regressions and ensure functionality throughout the migration.
- Use Spring’s Migration Guides
Refer to the official Spring Boot migration guide for detailed instructions.
- Monitor Performance
Compare performance metrics before and after migration to evaluate the impact of changes.
Conclusion
Migrating to Spring Boot 3 is an opportunity to modernize your application, improve performance, and align with the latest Java standards. While the migration process requires careful planning, the benefits—native image support, observability enhancements, and future-proof architecture—are well worth the effort.
By following the steps and best practices outlined in this guide, you can ensure a smooth transition to Spring Boot 3 and unlock the full potential of this powerful framework.
That's all for this tutorial, I hope you enjoyed it, and until our next tutorial, happy uploading and take care.
Thank you!