How to Dockerize a React Application
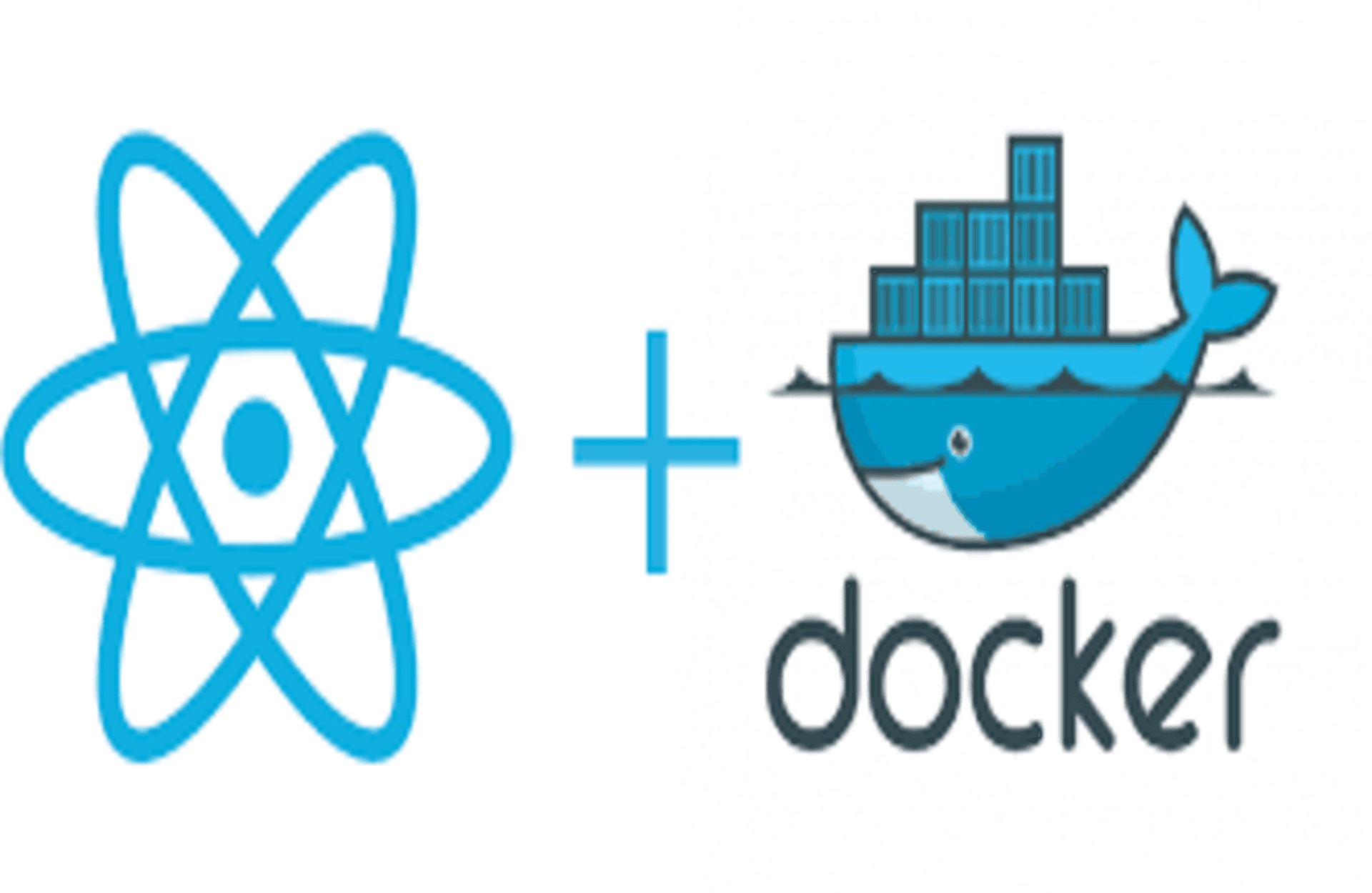
Content Table
- Prerequisites
- Create a Dockerfile
- Use an official Node.js runtime as a base image
- Set the working directory in the container
- Copy package.json and package-lock.json to the container
- Install dependencies
- Copy the rest of the application code to the container
- Build the React app
- Expose the port that the app will run on
- Define the command to run your app
- Create a `.dockerignore` File
- Build the Docker Image
- Run the Docker Container
Dockerization has become an essential practice in modern software development, offering numerous benefits such as easier deployment, increased scalability, and improved code consistency. React, being one of the most popular JavaScript libraries for building user interfaces, can greatly benefit from being dockerized.
Dockerizing a React application involves creating a Docker image that contains your React app and its dependencies. This allows you to package your application into a portable container, making it easy to deploy and run consistently across different environments.
In this tutorial we will discover the step-by-step how to Dockerize a React application.
Prerequisites
To Continue this tutorial, there are a some prerequisites that you need to fulfill:
- Docker Installed: Make sure Docker is installed on your machine. You can download Docker from the official website.
- React App: Have a React application ready. If you don't have one, you can quickly create a new React app using create-react-app:
npx create-react-app my-react-app
cd my-react-app
For more information please check the official website
Create a Dockerfile
In order to Dockerize your React application, you will need to create a Dockerfile. This file contains the instructions that Docker will use to build the container image for your application.
To create a Dockerfile, you will need to specify a base image. The base image you choose will depend on the requirements of your application. For a React application, a good base image to start with is the official Node.js image. This image includes all the necessary tools and dependencies to run a Node.js application.
Once you have chosen a base image, you can then specify the instructions for building and running your application. This may include copying your application's source code into the container, installing any necessary dependencies, and setting the appropriate environment variables.
### Use an official Node.js runtime as a base image
FROM node:17-alpine
### Set the working directory in the container
WORKDIR /app
### Copy package.json and package-lock.json to the container
COPY package*.json ./
### Install dependencies
RUN npm install
### Copy the rest of the application code to the container
COPY . .
### Build the React app
RUN npm run build
### Expose the port that the app will run on
EXPOSE 3000
### Define the command to run your app
CMD ["npm", "start"]
Create a .dockerignore
File
Create a file named .dockerignore to specify files and directories that should be ignored when copying files into the Docker image. This helps reduce the size of the image:
node_modules
build
.dockerignore
Dockerfile
Build the Docker Image
Building a Docker image is the next step in Dockerizing your React application. With the Dockerfile we created in the previous section, we can now build the container image. To build the Docker image, open the terminal and navigate to the root folder of your React application. Once you are in the correct directory, run the following command:
docker build -t <your-image-name> .
Replace <your-image-name>
with a name of your choice to identify your container image. The .
at the end of the command represents the current directory and tells Docker to look for the Dockerfile in the current directory.
Docker will now start building the image based on the instructions provided in the Dockerfile
. This process might take a few minutes, depending on the size of your application and the dependencies required.
Run the Docker Container
Now that you have successfully built the Docker image for your React application, it's time to run it as a container and access your application.
To run the Dockerized React application, open the terminal and run the following command:
docker run -d -p <host-port>:<container-port> <image-name>
Replace <host-port>
with the port on your host machine that you want to map to the container's port. Replace <container-port>
with the port on which your React application is running inside the container. Also, replace <image-name>
with the name you provided when building the Docker image.
The -d
flag runs the container in detached mode, meaning it runs in the background. This allows you to continue using the terminal.
After running the command, Docker will start the container and you will be able to access your React application by opening a web browser and navigating to http://localhost:<host-port>
.
Here's an example of a command with parameters already filled in.
docker run -d -p 3000:3000 my-react-app
This command maps port 3000 on your machine to port 3000 in the Docker container. Open your browser and navigate to http://localhost:3000 to view your React app running inside the Docker container.
In summary, Dockerizing a React application involves creating a Dockerfile, specifying dependencies, building the app, and running it in a Docker container. This approach simplifies deployment and ensures consistent behavior across different environments. Remember to customize the Dockerfile based on your specific project requirements.
That's all for this tutorial, I hope you enjoyed it, and until our next tutorial, take care.
Thank you!