Access AWS Lambda environment variables
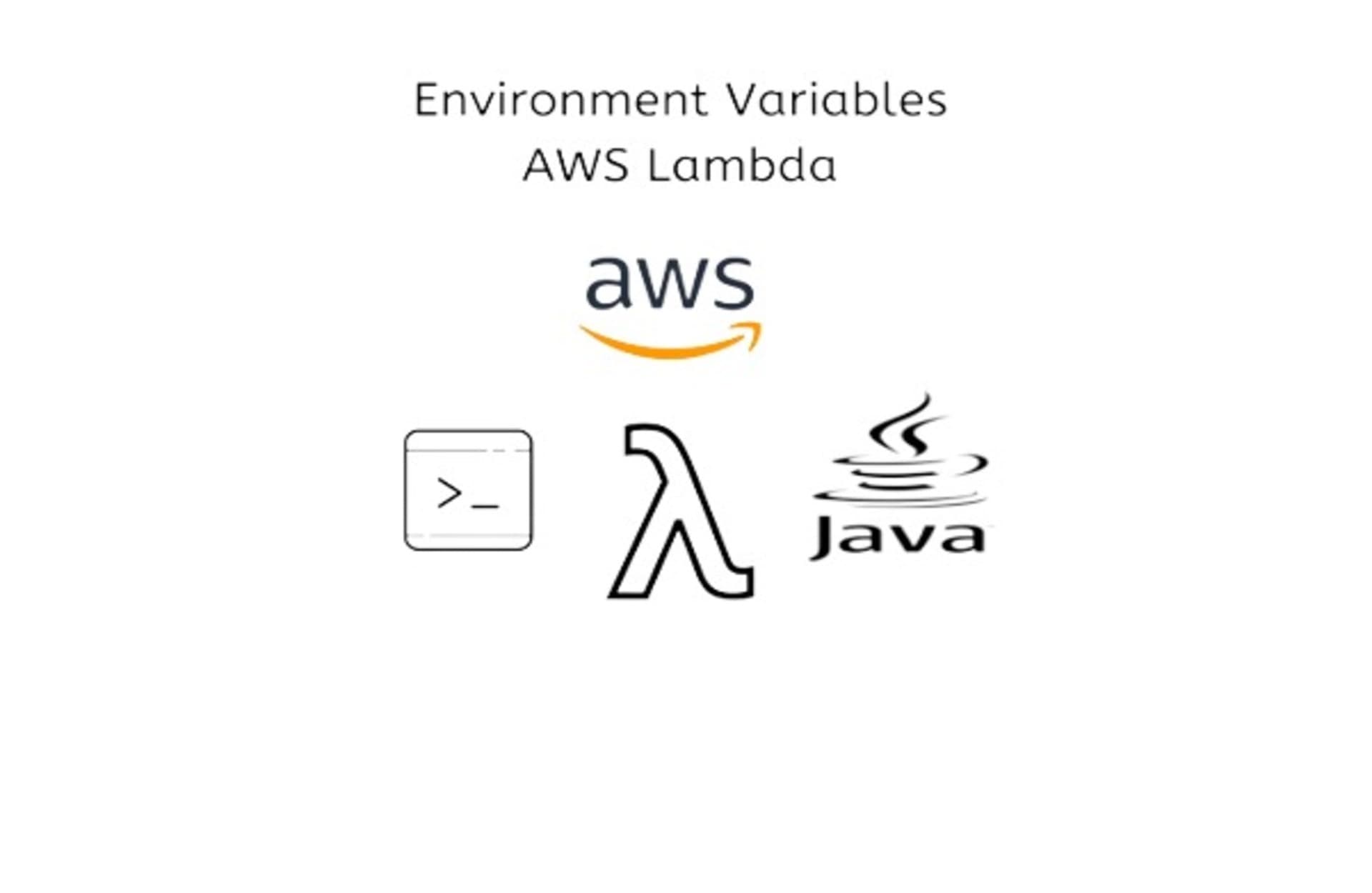
Lambda is a serverless computing service offered by Amazon Web Services (AWS) that allows you to run code without provisioning or managing servers. Java is a popular programming language among developers due to its versatility and extensive libraries. In this article, we will explore the methods for accessing environment variables for AWS Lambda functions developed using Java.
Accessing environment variables in AWS Lambda is a crucial aspect of developing robust Java applications. Environment variables allow developers to configure and customize Lambda functions without modifying the code. AWS Lambda provides a flexible and secure way to manage environment variables, giving developers full control over their applications' behavior.
Prerequisites
To Continue this tutorial, there are a some prerequisites that you need to fulfill:
- An AWS account to configure Your Lambda function.
- Install JDK 11 or higher
- Java Editor (Intellij or Eclipse)
Add Environment Variables In AWS Lambda Using AWS Console
We can add an environment variable via the AWS console.
First, you need to log in to the AWS Management Console. If you don't have an account, you can sign up to create one.
Next, navigate to Lambda
-> Functions
-> Your Lambda
-> Configuration
-> Environment variables
-> and then click on Edit
button to add new environment variable.
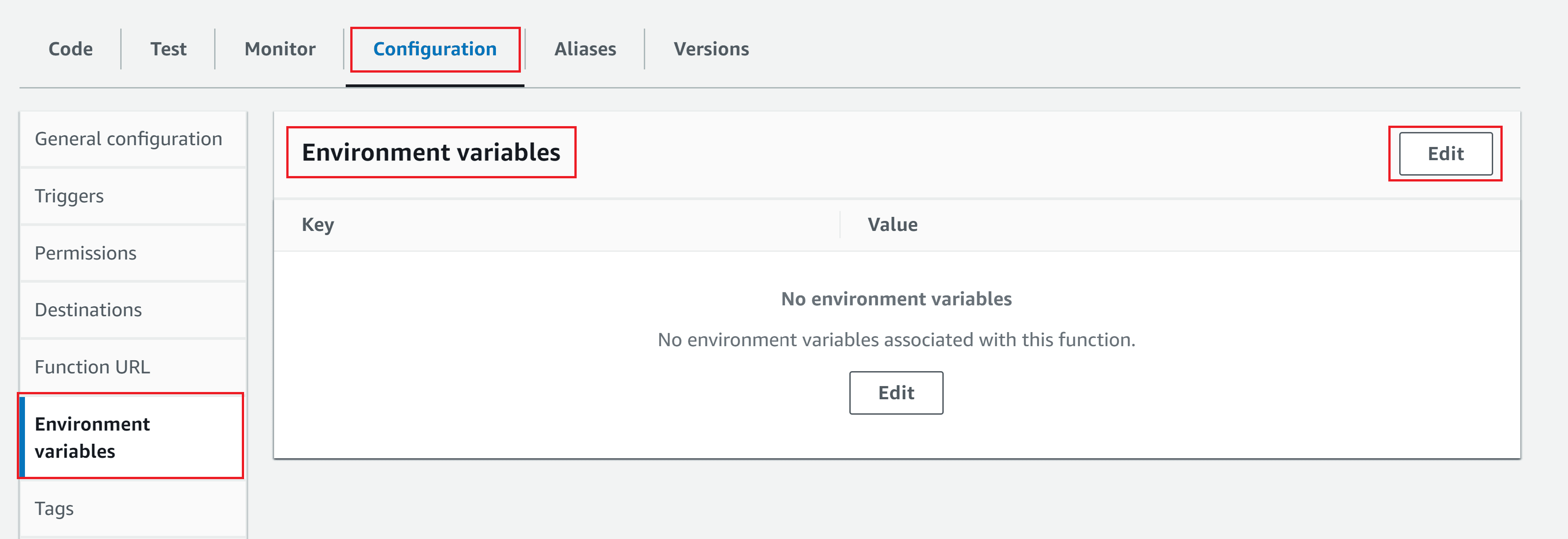
When you click on the Edit
button, the screen below appears to add a new variable. After adding the new environment variable, click on the Save
button.
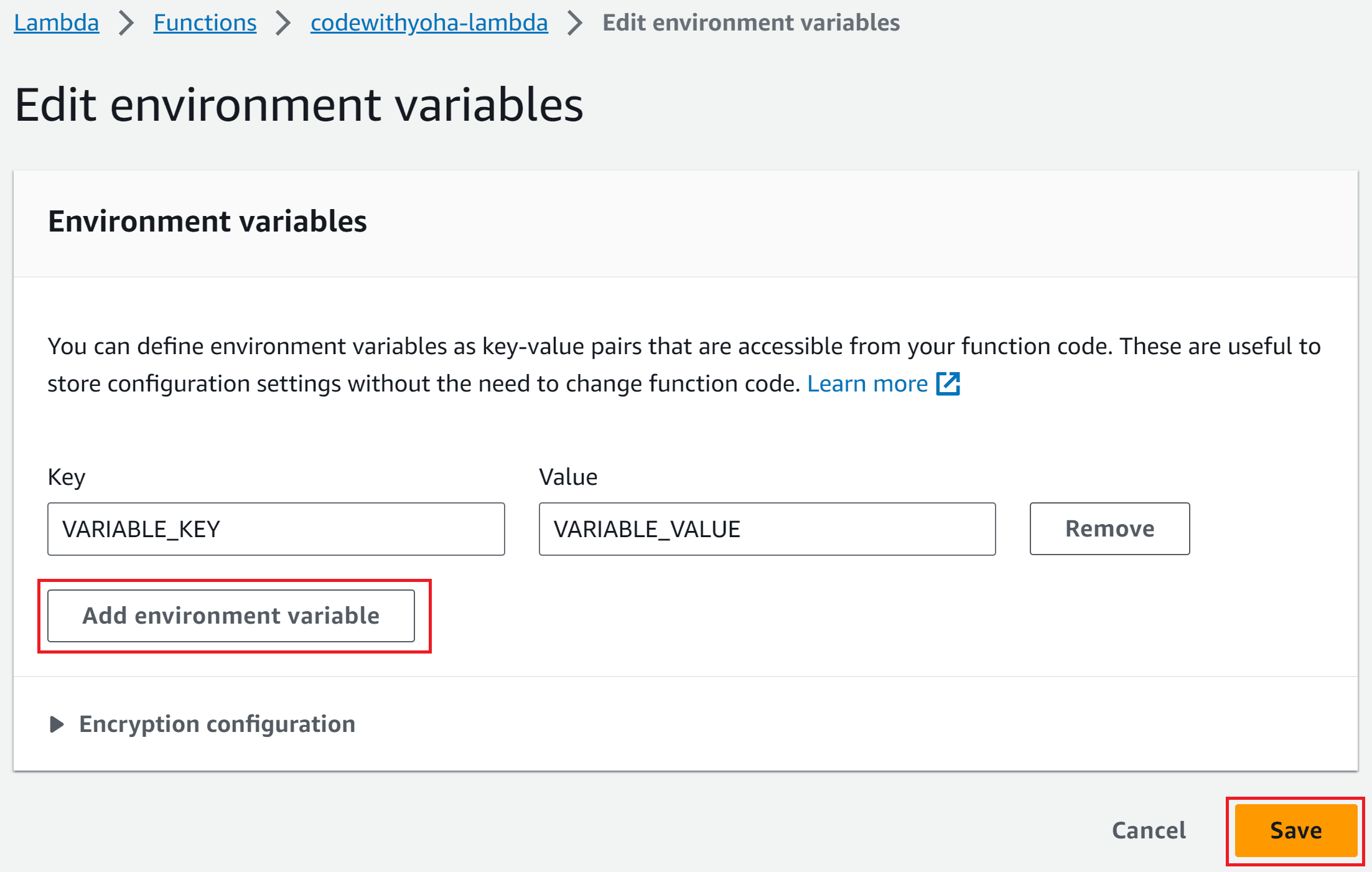
You can define environment variables as key-value pairs that are accessible from your function code.
PS: Follow this Tutorial to create your first AWS Lambda function if you don't already have one.
Accessing Environment Variables in Java Lambda Functions
To access environment variables in your Java Lambda function, you can use System.getenv()
.
Here's an example of how you can access an environment variable called VARIABLE_KEY
in your Lambda function:
public class MyLambdaHandler implements RequestHandler<SomeInputType, SomeOutputType> {
@Override
public SomeOutputType handleRequest(SomeInputType input, Context context) {
// Access environment variables
String variableValue = System.getenv("VARIABLE_KEY");
// Your Lambda function logic here
return someOutput;
}
}
Replace VARIABLE_KEY
with the name of the environment variable you want to access.
Remember to replace SomeInputType
and SomeOutputType
with the appropriate data types for your Lambda function. The Context object can be useful for additional context and information about the Lambda execution, but you can omit it if you don't need it.
That's all for this tutorial, I hope you enjoyed it, and until our next tutorial, take care.
Thank you!